简介
熟练使用各种网络请求技术对前端工程师来说是一项必需的技能,现在各种网络请求工具层出不穷,从原生的HttpRequest到经历各种封装的库如 Ajax,SuperAgent,Fly 等等。今天我们来聊聊 Axios
axios 是什么
axios 是一个基于promise 的http库,可以用在浏览器与nodejs中,是当今流行的一个框架
axios 使用
下载依赖
初次使用需要下载axios依赖包
npm install axios
请求
get请求
- 首先是无参请求
1 2 3 4 5 6 7
| axios.get('后台接口api') .then(response=>{ consolg.log(response) }) .catch(error=> { console.log(error); });
|
使用.then方法来接收响应体,使用.catch方法来处理异常
- 有参请求
1 2 3 4 5 6 7 8 9 10 11 12
| axios.get('后台接口api', { params: { id: 1, name:'咸鱼' } }) .then(response=>{ console.log(response); }) .catch(error=>{ console.log(error); });
|
post请求
post
1 2 3 4 5 6 7 8 9 10
| axios.post('后台接口api', { id: 1, name: 'fish' }) .then(response=>{ console.log(response); }) .catch(error=>{ console.log(error); });
|
整合
个人喜欢且推荐参数全部整合起来,类似报文的方式发起请求
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| axios({ url:'api', method:'post|get', headers:{键值对}, data:{ name:'fish' } }) .then(response=>{ console.log(res) }) .catch(error=>{ console.log(error) })
|
发起请求后我们就可以在开发者调试工具上看到我们的请求参数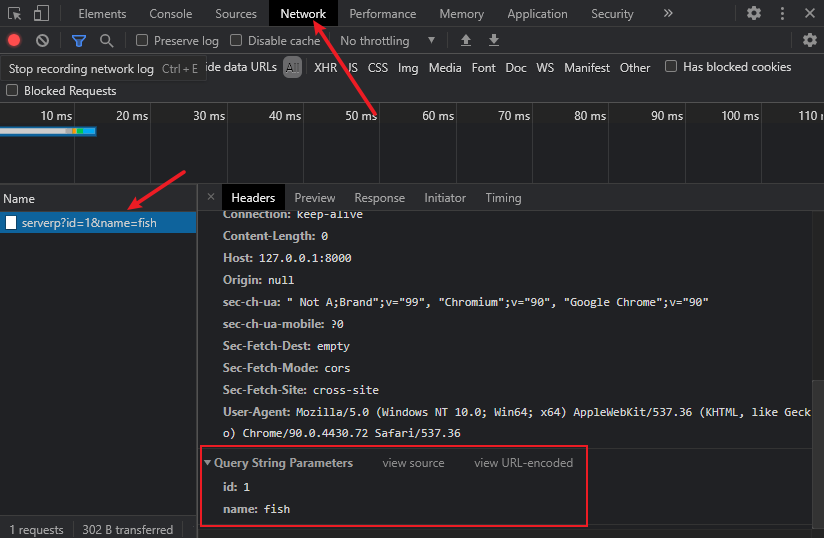
并发
有时候我们需要同时发起多个不同的请求,这时我们就可以使用并发
1 2 3 4 5 6 7 8 9 10 11 12 13
| axios.all([ axios.post('后台api',"name:fish"), axios.get('后台api',{parms:{id:1}}) ]) .then( axios.spread((res1,res2)=>{ console.log(res1) console.log(res2) }) ) .catch(error=>{ console.log(error) })
|
全局配置
在开发中,会经常遇到同一个页面多个地方请求同一个url,多次使用同一个url时我们可以将那个url提取出来,做一个全局配置
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| axios.default.method = 'GET'|'POST'
axios.default.baseURL = '后台api'
axios.default.params={id:1,name:'fish'}
axios.defaults.timeout = 5000
axios.get('test') .then(res=>{ console.log(res) }) .catch(error=>{ console.log(error) })
|
配置实例
适用于配置多个请求
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| const req_1 = axios.create({ baseURL:'后台api', timeout:5000 })
req_1({ url:'test' }) .then(res=>{ console.log(res) }) .catch(error=>{ console.log(error) })
|
拦截器
适用于在请求发起前或响应时,进行相关操作,常用于强制登录,处理响应信息等等
1 2 3 4 5 6 7 8 9 10 11
| axios.interceptors.request.use( config=>{ console.log('进入了拦截器') retrun config }, err=>{ } )
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| axios.interceptors.response.use( config=>{ console.log('进入拦截器')
config.data.name = '张三'
retrun config.data }, error=>{ } )
|
当定义了多个拦截器时 执行顺序是:
请求拦截器是谁在定义的最后,谁先拦截,相应拦截器则是谁先定义谁先拦截。
总结
axios 是一个强大的http库 依赖于原生的 ES6 Promise
通常内置在vue中,能极大提高开发效率